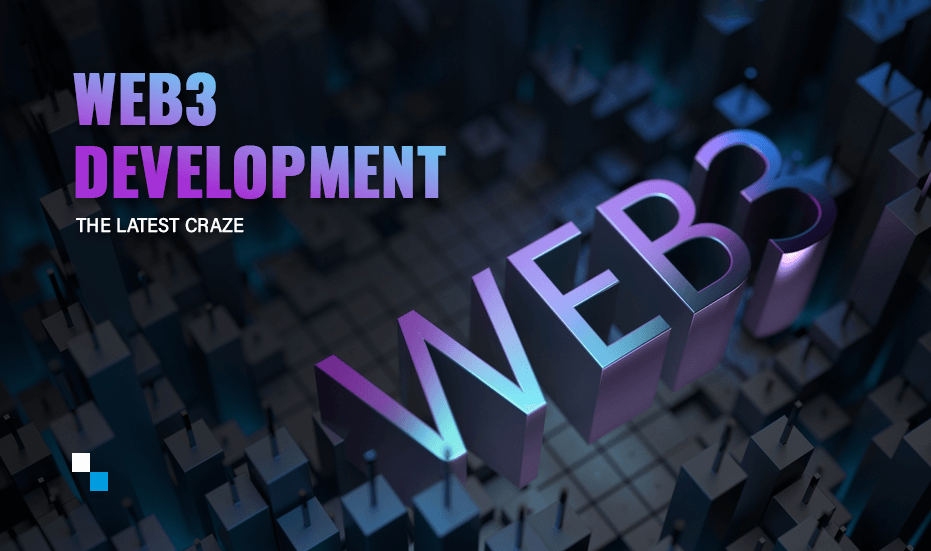
The world of decentralized applications (dApps) is rapidly evolving, with the rise of Web3 technologies reshaping how applications and services are built, deployed, and used. Decentralization aims to remove intermediaries, granting users more control over their data, identities, and transactions. Web3 development brings this vision to life, providing developers with a new paradigm of building applications that are more transparent, secure, and resistant to censorship.
In this article, we’ll dive deep into what Web3 development is, how decentralized apps (dApps) work, and the frameworks and tools you can use to build them. Whether you’re an experienced developer or just getting started, this guide will provide practical insights into making Web3 development simple and accessible.
What is Web3 Development?
At its core, Web3 is the next iteration of the internet. Web1, the original internet, was a read-only web where users could access information but couldn’t interact with it in meaningful ways. Web2 introduced interactivity, enabling social media platforms, e-commerce, and user-generated content. However, Web2 is still controlled by large centralized corporations, resulting in issues like data privacy concerns, lack of user control, and potential censorship.
Web3 flips this model on its head by using blockchain technology to create a decentralized web. This means no central authority governs the internet, and individuals have ownership of their data. Instead of relying on intermediaries like banks, social networks, or content providers, Web3 uses decentralized networks and protocols to enable peer-to-peer interactions directly.
A key component of Web3 is decentralized applications, or dApps. These are applications that run on decentralized networks like Ethereum, Solana, and others, instead of traditional centralized servers. These applications typically interact with smart contracts to ensure transparent and secure transactions.
What Are dApps?
Decentralized applications (dApps) are the backbone of Web3. They leverage blockchain technology to provide services in a decentralized manner, giving users control over their interactions, data, and digital assets. Unlike traditional apps, dApps are not stored on a central server but instead operate on a blockchain, which is a distributed ledger. This provides several key advantages:
- Decentralization: No single entity controls the application. Power is distributed across a network of nodes.
- Transparency: All transactions and actions within a dApp are recorded on the blockchain, making them auditable and transparent.
- Security: Due to the immutable nature of blockchains, once data is recorded, it cannot be tampered with.
- Trustlessness: dApps don’t require users to trust a central authority. Instead, the blockchain and smart contracts enforce the rules.
- Ownership: Users retain control over their data and assets within dApps, without relying on intermediaries.
Examples of dApps include decentralized finance (DeFi) platforms, decentralized exchanges (DEXs), non-fungible token (NFT) marketplaces, and gaming platforms. Building a dApp requires more than just creating a user interface; it involves integrating blockchain functionality, smart contracts, and often a decentralized storage system.
Building dApps: Key Components
Creating a decentralized application involves a few fundamental components:
- Smart Contracts: These are self-executing contracts where the terms of the agreement are written directly into code. They allow transactions and agreements to be made without intermediaries. Smart contracts are deployed on blockchain networks such as Ethereum or Binance Smart Chain (BSC).
- Blockchain Network: The decentralized ledger that records all transactions and states within the application. Popular blockchain networks for dApp development include Ethereum, Polkadot, Solana, and others.
- Frontend Development: The user-facing part of the dApp is similar to any web application but needs to interact with the blockchain. This requires libraries and frameworks that facilitate communication with blockchain networks, such as Web3.js or Ethers.js.
- Wallet Integration: dApps require a way for users to interact with their cryptocurrencies and digital assets. This is where Web3 wallets like MetaMask, Trust Wallet, or Coinbase Wallet come in, enabling users to sign transactions and manage their tokens.
- Decentralized Storage: Since dApps need to store data off-chain (outside of the blockchain), decentralized storage solutions like IPFS (InterPlanetary File System) and Arweave are commonly used to store media, documents, and other data associated with the app.
Frameworks and Tools for dApp Development
To build a dApp, developers rely on various frameworks and tools that simplify the integration of smart contracts, blockchain interactions, and the overall development process. Here are some of the most popular frameworks and tools used for Web3 development:
1. Truffle Suite
Truffle is one of the most popular development environments for Ethereum-based dApps. It provides a comprehensive suite of tools that make building and testing smart contracts easier:
- Truffle Framework: A powerful and flexible development framework for Ethereum dApps, it simplifies writing, testing, and deploying smart contracts.
- Truffle Boxes: Pre-configured templates that help developers quickly get started with different types of dApp projects.
- Ganache: A personal Ethereum blockchain that developers can use to test smart contracts locally before deploying them to a live network.
- Drizzle: A front-end library that helps to easily integrate a React-based interface with blockchain data.
2. Hardhat
Hardhat is another Ethereum-focused development environment that provides a set of powerful tools for developers:
- Smart Contract Compilation: Hardhat helps developers compile and test smart contracts efficiently.
- Local Blockchain Environment: Like Ganache, Hardhat provides an in-memory blockchain to test smart contracts.
- Plugins: Hardhat has an extensive plugin ecosystem, making it easy to integrate additional functionalities, such as deploying contracts or interacting with Ethereum Virtual Machine (EVM) networks.
- Debugging Tools: Hardhat provides advanced debugging tools to help developers identify and fix issues in their smart contract code.
3. Alchemy
Alchemy is a powerful blockchain development platform that provides a set of tools for building, monitoring, and scaling blockchain applications. It supports Ethereum, Polygon, and other networks, offering developers easy access to blockchain data. Alchemy’s tools allow for building dApps with better scalability, real-time data streaming, and advanced monitoring capabilities.
4. Moralis
Moralis is a backend-as-a-service (BaaS) platform for Web3 developers. It simplifies the development of dApps by offering pre-built solutions for user authentication, real-time database syncing, and blockchain event tracking. Moralis supports Ethereum, Binance Smart Chain, and other networks, reducing the complexity of dApp development by providing backend services out-of-the-box.
5. Web3.js & Ethers.js
These two JavaScript libraries are essential tools for integrating frontend applications with blockchain networks:
- Web3.js: The original JavaScript library for interacting with the Ethereum blockchain, Web3.js makes it easy to communicate with smart contracts, send transactions, and manage blockchain data.
- Ethers.js: A lightweight alternative to Web3.js, Ethers.js focuses on providing a secure and user-friendly experience for developers. It is especially popular in the Ethereum ecosystem and is often used for managing wallet connections and transactions.
Developing a Simple dApp: A Step-by-Step Guide
Now that you understand the core components and tools involved in building a dApp, let’s walk through a simplified version of the development process. In this example, we’ll build a simple Ethereum-based voting dApp that allows users to vote on a topic.
1. Set Up Your Development Environment
- Install Node.js, NPM, and Git.
- Install Truffle or Hardhat as your development framework.
- Set up a test network like Ganache or use a testnet like Rinkeby for testing purposes.
- Install Web3.js or Ethers.js to interact with the Ethereum blockchain.
2. Write the Smart Contract
- Create a new Truffle or Hardhat project.
- Write the smart contract to define the rules of your voting system. For example, users can vote on a specific issue, and the smart contract will tally the votes.
solidityCopy codepragma solidity ^0.8.0;
contract Voting {
mapping(address => bool) public voters;
mapping(string => uint256) public votes;
function vote(string memory candidate) public {
require(!voters[msg.sender], "You have already voted.");
votes[candidate]++;
voters[msg.sender] = true;
}
function getVotes(string memory candidate) public view returns (uint256) {
return votes[candidate];
}
}
3. Deploy the Smart Contract
- Use Truffle or Hardhat to deploy the smart contract to a test network.
- Once deployed, the contract address will allow you to interact with it from your frontend.
4. Build the Frontend
- Set up an HTML and JavaScript frontend that communicates with the Ethereum blockchain using Web3.js or Ethers.js.
- Create a simple interface with buttons to vote and check vote counts.
5. Connect to a Wallet
- Integrate a Web3 wallet like MetaMask to enable users to sign transactions and interact with your dApp.
6. Test and Deploy
- Test the dApp on a testnet.
- Once everything works correctly, deploy the application on the mainnet.
Conclusion
Web3 development and dApp creation can seem overwhelming, but with the right frameworks and tools, it becomes much more approachable. The decentralized nature of Web3 applications offers many exciting opportunities for developers to build innovative solutions that give users more control over their digital lives.
By mastering tools like Truffle, Hardhat, Web3.js, and others, developers can easily dive into building decentralized applications that work on a global, censorship-resistant scale. Whether you’re building a DeFi platform, NFT marketplace, or a simple voting app, the possibilities in Web3 are vast and ever-expanding.