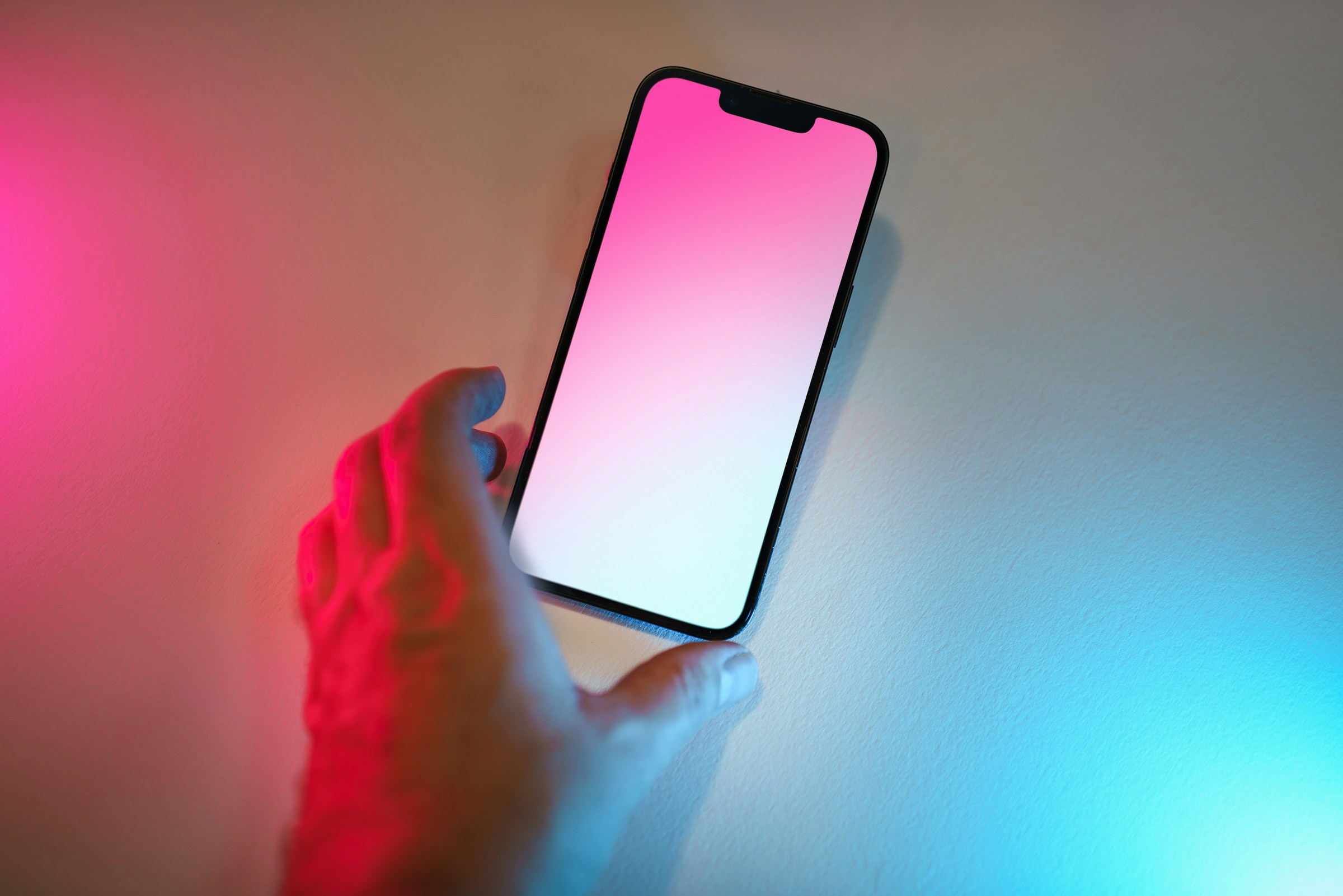
Mobile apps have become an integral part of our daily lives, driving everything from communication and productivity to entertainment. If you’re someone who’s fascinated by mobile app development but has no idea where to start, then Flutter is an excellent choice. This guide will walk you through everything you need to know about building your first app using Flutter, a powerful and popular framework from Google that simplifies the app development process.
Why Choose Flutter?
Before diving into the development process, it’s essential to understand why Flutter is such a great choice for beginners. Flutter offers several advantages, including:
- Cross-Platform Development: Write a single codebase and run it on both iOS and Android, saving time and effort.
- Rich UI Components: Flutter’s widget system allows you to build beautiful, customizable user interfaces with ease.
- High Performance: Built on Dart and powered by a native engine, Flutter apps are smooth and responsive.
- Large Community & Resources: There are countless tutorials, guides, and plugins available, making it easier for beginners to learn.
- Hot Reload: This feature allows you to see changes in real-time as you code, which speeds up the development and debugging process.
Now that we understand why Flutter is beneficial, let’s dive into building your first app.
Step 1: Setting Up Your Environment
Before you start coding, you’ll need to set up your development environment.
Install Flutter
- Download Flutter SDK: Visit flutter.dev and download the Flutter SDK for your operating system (Windows, macOS, or Linux).
- Unzip the File: Extract the downloaded Flutter SDK file into a location on your computer.
- Set Path: To use Flutter from the command line, add Flutter to your system path. This varies depending on your OS:
- Windows: Open the System Properties > Environment Variables > Edit the Path variable and add the Flutter SDK path.
- macOS: Open your terminal and edit the
~/.bash_profile
(or~/.zshrc
for newer systems) to include the Flutter path. - Linux: Edit your
~/.bashrc
to add the Flutter path.
- Run Flutter Doctor: Open your terminal or command prompt and type:Copy code
flutter doctor
This command will check whether you have all the necessary tools installed, like Dart, Android Studio, and Xcode (for iOS). Follow any instructions to install missing dependencies.
Install an IDE
While you can technically write Flutter code using any text editor, it’s best to use an Integrated Development Environment (IDE) like Visual Studio Code or Android Studio. Both of these IDEs have excellent support for Flutter.
- Visual Studio Code: Download and install VS Code, then install the Flutter and Dart extensions.
- Android Studio: Download and install Android Studio, then configure it with the Flutter plugin.
Step 2: Creating Your First Flutter App
Now that your environment is set up, it’s time to create your first Flutter app. Flutter makes this process simple by providing command-line tools to scaffold a new project.
- Open Terminal/Command Prompt and run:luaCopy code
flutter create my_first_app
This will create a new Flutter project in a folder namedmy_first_app
. - Navigate to the Project Directory:bashCopy code
cd my_first_app
- Open the Project in Your IDE: If you’re using VS Code, type:cssCopy code
code .
Project Structure
Your Flutter project will have a structure like this:
bashCopy codemy_first_app/
|- android/
|- ios/
|- lib/
|- main.dart
|- test/
|- pubspec.yaml
- android/ and ios/: These folders contain the platform-specific code for Android and iOS.
- lib/: This folder is where your Dart code lives.
main.dart
is the entry point of your app. - pubspec.yaml: This file is like a configuration file for your project, where you specify dependencies, assets, and more.
Step 3: Writing Your First Flutter Code
Open lib/main.dart
. You’ll see some boilerplate code that Flutter provides. Let’s break it down:
dartCopy codeimport 'package:flutter/material.dart';
void main() {
runApp(MyApp());
}
class MyApp extends StatelessWidget {
@override
Widget build(BuildContext context) {
return MaterialApp(
home: Scaffold(
appBar: AppBar(
title: Text('My First App'),
),
body: Center(
child: Text('Hello, World!'),
),
),
);
}
}
Here’s what this code does:
main()
: This is the entry point of your app. TherunApp()
function takes in your root widget and makes it the base of your app.MyApp
Class: This is your main app class, which is aStatelessWidget
. It returns aMaterialApp
, which is the top-level widget in Flutter that provides Material Design (a Google UI design system).- Scaffold: This is a basic layout structure in Flutter that provides a visual scaffold, including app bars, drawers, etc.
- AppBar and Text Widgets: The app bar displays the title “My First App,” and the body of the app displays the text “Hello, World!”.
Run the App
Now, let’s run your app.
- Connect a Device or Emulator: You can either connect a physical Android/iOS device or set up an Android emulator/iOS simulator in Android Studio or Xcode.
- Run the App:arduinoCopy code
flutter run
Within moments, your app will launch on the device/emulator, and you’ll see your first app displaying “Hello, World!”.
Step 4: Adding More Features
Now that you have the basic structure in place, let’s add a simple interactive feature: a button that increments a counter.
Replace the content inside Scaffold
with the following code:
dartCopy codeimport 'package:flutter/material.dart';
void main() => runApp(MyApp());
class MyApp extends StatelessWidget {
@override
Widget build(BuildContext context) {
return MaterialApp(
home: CounterWidget(),
);
}
}
class CounterWidget extends StatefulWidget {
@override
_CounterWidgetState createState() => _CounterWidgetState();
}
class _CounterWidgetState extends State<CounterWidget> {
int _counter = 0;
void _incrementCounter() {
setState(() {
_counter++;
});
}
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: Text('Counter App'),
),
body: Center(
child: Column(
mainAxisAlignment: MainAxisAlignment.center,
children: <Widget>[
Text(
'You have pressed the button this many times:',
),
Text(
'$_counter',
style: Theme.of(context).textTheme.headline4,
),
],
),
),
floatingActionButton: FloatingActionButton(
onPressed: _incrementCounter,
tooltip: 'Increment',
child: Icon(Icons.add),
),
);
}
}
Here’s what’s new:
- StatefulWidget: We introduced a
StatefulWidget
, which can change its state and update the UI in response to those changes. - Counter Logic:
_counter
is a variable that holds the current count. Every time the button is pressed,_incrementCounter()
is called, andsetState()
updates the UI. - FloatingActionButton: This is a circular button that triggers the
_incrementCounter()
method whenever pressed.
Run the App Again
When you run the app, you’ll now see a counter displayed, which increments every time you press the button.
Step 5: Customizing Your App
You can personalize your app further by:
- Changing Colors: Modify the
AppBar
color by addingbackgroundColor: Colors.blueGrey
in theAppBar
widget. - Adding New Widgets: Explore other widgets like
ListView
,GridView
,Card
,TextField
, and more. - Styling Text: Customize the
Text
widget by modifying itsstyle
property with customTextStyle
.
Step 6: Testing and Debugging
Testing is crucial in app development, especially as apps grow more complex. Flutter provides built-in support for testing at three levels:
- Unit Tests: Test individual functions, methods, or classes.
- Widget Tests: Test individual UI components.
- Integration Tests: Test the complete app.
You can write tests in the test/
directory. For example, here’s a simple unit test for the counter logic:
dartCopy codevoid main() {
test('Counter increments', () {
var counter = Counter();
counter.increment();
expect(counter.value, 1);
});
}
Conclusion
Building your first mobile app with Flutter is an exciting journey, and with the framework’s simplicity, it becomes a more accessible experience for beginners. Flutter’s comprehensive widget library, cross-platform capabilities, and excellent performance make it a go-to choice for modern mobile development.
Once you get comfortable with the basics, explore more advanced topics like state management (e.g., Provider
or Bloc
), animations, or integrating third-party libraries. The sky’s the limit in what you can create with Flutter!